1. Modules
The Comprehensive Perl Archive Network -CPAN- (
https://metacpan.org) provides more than 100,000 Perl modules. A module is a set of related functions in a library file. This file is reusable by other modules or programs. Most modules are written in Perl and can be invoked without problems.
1.1 Find and install a module
Modules have to be installed. Instructions on installing can be find on CPAN. Let's follow the necessary steps.
Step 1: go to
https://metacpan.orgStep 2: I'm interested in a string trimming function. Enter 'Trim' in the search field of the search engine:
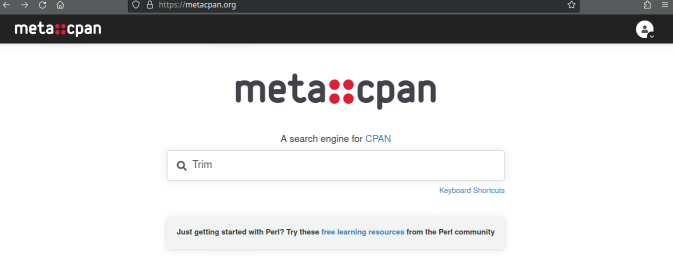
Press Enter an you'll see something like this:
Step 3: Search for
Text::Trim - remove leading and/or trailing whitespace from strings and click the link. The result page is:
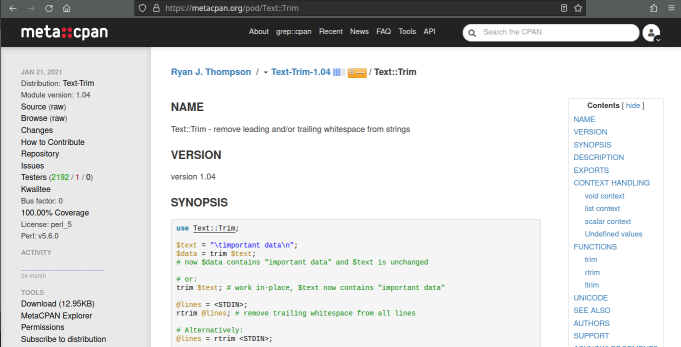
On the left side, you find Install instructions. I recommend installing via
cpanm Text::Trim; # cpanm has to be installed first of course: cpan App::cpanminus
1.2 How to use?
In the text under 'Functions' you'll find the available functions: trim, rtrim and ltrim and a short description. Examples on how to use the module and its functions are presented. One example:
#!/usr/bin/perl
use strict;
use warnings;
use Text::Trim; # invoke the module Text::Trim
my $text = " important data ";
my $trimmed_text = trim($text);
print("-$text-|-$trimmed_text-\n");
The output will be:
- important data -|-important data-
1.3 Error?
Maybe you get an error message like "Can't locate Text/Trim.pm in @INC ...". You can solve the problem by adding the path of the .pm file to the PERL5LIB environment variable. In my case where Text/Trim.pm is located in /home/reinier/perl5/lib/perl5/I should write the following:
export PERL5LIB=/home/reinier/perl5/lib/perl5/
1.4 Uninstall
You can uninstall a module via
cpanm --uninstall Text::Trim;
1.5 Why reinvent the wheel?
The previous example is probably not convincing. You could easily find a regex solution:
#!/usr/bin/perl
use strict;
use warnings;
my $text = " important data ";
$text =~ s/^\s+//
$text =~ s/\s+$//
print("-$text-\n");
But what if you what to make permutations of an array of variable length? That's really time consuming if you want to make your own solution. So, search on CPAN and find a module. Example of one solution:
#!/usr/bin/perl
use strict;
use warnings;
use Algorithm::FastPermute ('permute');
my @array = (1..3);
permute { print "@array\n"; } @array;